What is triple equals in JavaScript?
In this article, we'll explore the triple equals operator (===) in JavaScript, its purpose, and how it compares to the double equals operator (==).
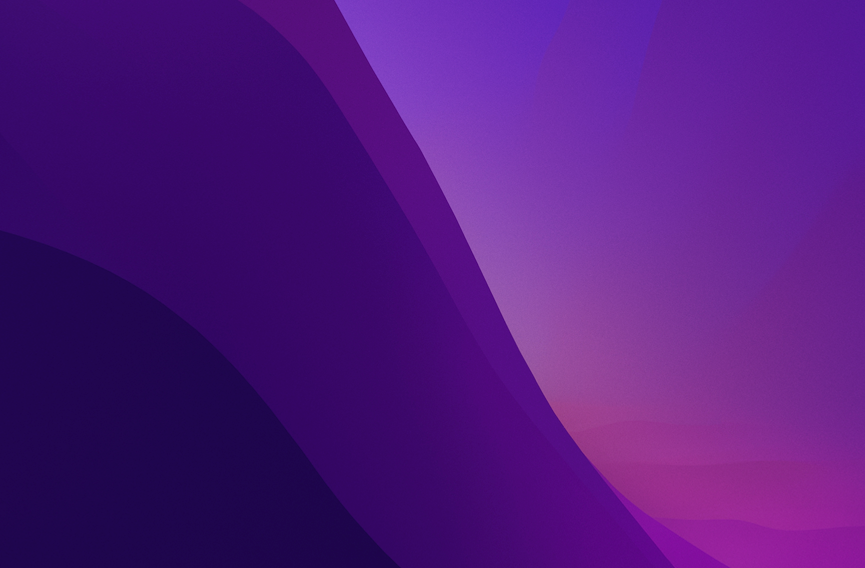
What is Triple Equals in JavaScript?
The triple equals operator (===) in JavaScript is a strict equality comparison operator that checks both value and type equality. Unlike its more lenient cousin, the double equals operator (==), triple equals doesn't perform type coercion before making the comparison. This makes it more predictable and generally safer to use in your code.
1// Double equals (==) with type coercion2console.log(5 == "5"); // true3console.log(1 == true); // true45// Triple equals (===) without type coercion6console.log(5 === "5"); // false7console.log(1 === true); // false
In this example, we can see how double equals (==) performs type coercion, converting the string "5" to a number before comparison. Triple equals (===), however, checks both value and type, resulting in false when comparing different types.
The callback function takes the current element as its parameter (num in this case) and returns true if the number is even (num % 2 === 0). Filter creates a new array containing only the elements for which the callback returned true.
💡 Pro tip: Always use triple equals (===) by default unless you have a specific reason to use double equals (==). This helps prevent unexpected behavior in your code.
1// Examples of JavaScript type coercion2console.log(0 == false); // true (coerced)3console.log(0 === false); // false (strict)4console.log("" == false); // true (coerced)5console.log("" === false); // false (strict)6console.log([1,2] == "1,2"); // true (coerced)7console.log([1,2] === "1,2"); // false (strict)
Let's break down what happens during type coercion:
-
When using double equals (==):
- JavaScript tries to convert operands to the same type
- This can lead to unexpected results
- The rules for coercion are complex and hard to remember
-
When using triple equals (===):
- No type conversion occurs
- Both value and type must match exactly
- Results are more predictable and easier to reason about
For example:
1// Type coercion examples2null == undefined // true3null === undefined // false451 == true // true61 === true // false780 == "" // true90 === "" // false
Triple equals should be your default choice for comparisons in JavaScript because:
- It's more predictable
- It catches type-related bugs early
- It makes code intentions clearer
- It's generally faster (no type coercion needed)
Let's explore some real-world examples where triple equals is crucial.
Example 1 - Basic Comparisons
Let's look at a real-world scenario where type comparison really matters. Imagine you're building a website that checks if a user meets an age requirement. The user's age might come from one source as a number, while the age limit might come from another source (like an API) as a string.
1// Basic value comparisons2const userAge = 25;3const ageLimit = "25";45// Double equals - potentially dangerous6if (userAge == ageLimit) {7 console.log("Equal with =="); // This will execute8}910// Triple equals - safer11if (userAge === ageLimit) {12 console.log("Equal with ==="); // This won't execute13}1415// Best practice: Convert types explicitly if needed16if (userAge === parseInt(ageLimit)) {17 console.log("Equal after conversion"); // This will execute18}
Example 2 - Comparing Different Types
Here's where things get interesting! Let's explore how JavaScript handles comparisons between different types like numbers, booleans, and empty strings. This is super important because these situations come up all the time when dealing with form inputs or API responses.
1// Comparing different data types2const value = 0;3const falsy = false;4const empty = "";56console.log(value == falsy); // true7console.log(value === falsy); // false8console.log(empty == falsy); // true9console.log(empty === falsy); // false1011// Real-world example12function checkValue(input) {13 // Better than input == false14 return input === false;15}
Example 3 - Common Gotchas
Now, let's tackle some tricky situations that often trip up even experienced developers. We'll see how JavaScript handles comparisons between numbers, strings, arrays, and objects - and why using === can save you from some real head-scratchers!
1// Common comparison pitfalls2const number = 0;3const string = "0";4const array = [0];5const object = new Number(0);67console.log(number == string); // true8console.log(number === string); // false910console.log(number == array); // true11console.log(number === array); // false1213console.log(number == object); // true14console.log(number === object); // false
Example 4 - Null and Undefined
Here's a classic JavaScript puzzle: dealing with null and undefined. These two values can cause some serious bugs if you're not careful. Let's see how triple equals helps us handle them properly and write more reliable code.
1// Handling null and undefined2let nullValue = null;3let undefinedValue;45console.log(nullValue == undefined); // true6console.log(nullValue === undefined); // false78// Best practice for checking null/undefined9function isNullOrUndefined(value) {10 return value === null || value === undefined;11}
Example 5 - Objects and Arrays
Last but definitely not least, let's dive into comparing objects and arrays. This is where a lot of developers get caught off guard! You might think two objects with the same content are equal, but JavaScript has other ideas. Here's what you need to know:
1// Object and array comparisons2const obj1 = { value: 1 };3const obj2 = { value: 1 };4const obj3 = obj1;56console.log(obj1 === obj2); // false (different references)7console.log(obj1 === obj3); // true (same reference)89// Arrays10const arr1 = [1, 2, 3];11const arr2 = [1, 2, 3];12console.log(arr1 === arr2); // false (different references)1314// Comparing array contents15console.log(JSON.stringify(arr1) === JSON.stringify(arr2)); // true
Practice Questions
Below are some practice coding challenges to help you master the triple equals operator in JavaScript.
JavaScript Type Coercion: What Happens When Adding String '5' and Number 3
JavaScript Comparison: Understanding the Difference Between == and === Operators
JavaScript Type Coercion Risk in Payment Processing: Loose Equality vs Strict Comparison
Additional Resources
Common Interview Questions
- What's the difference between == and ===?
- When might you want to use == instead of ===?
- How does type coercion work in JavaScript?
Remember: When in doubt, use triple equals (===) for safer, more predictable code!
Learn to code, faster
Join 660+ developers who are accelerating their coding skills with TechBlitz.
Read related articles
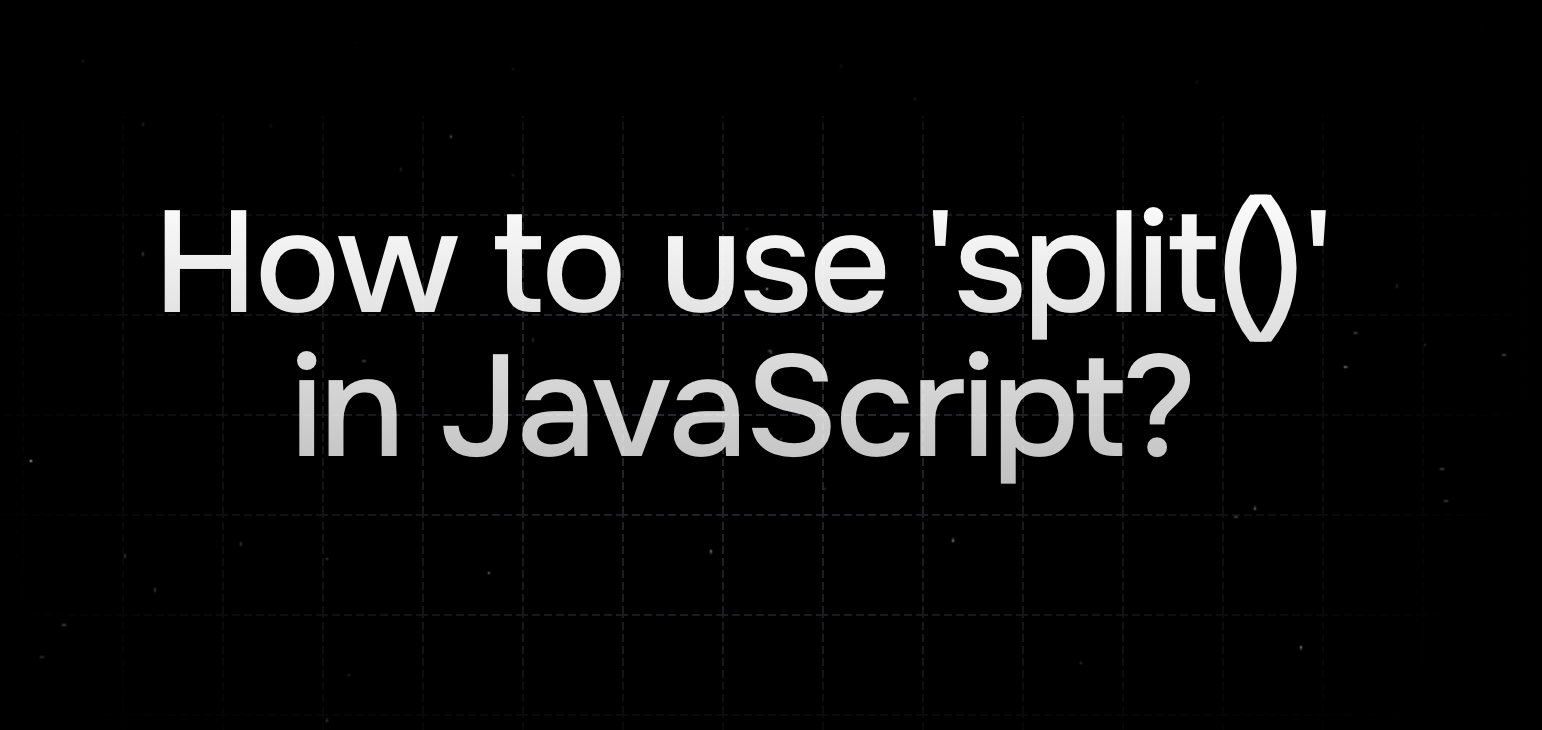
How to use split() in JavaScript
Understand how to use split() in JavaScript to break strings into arrays fo...
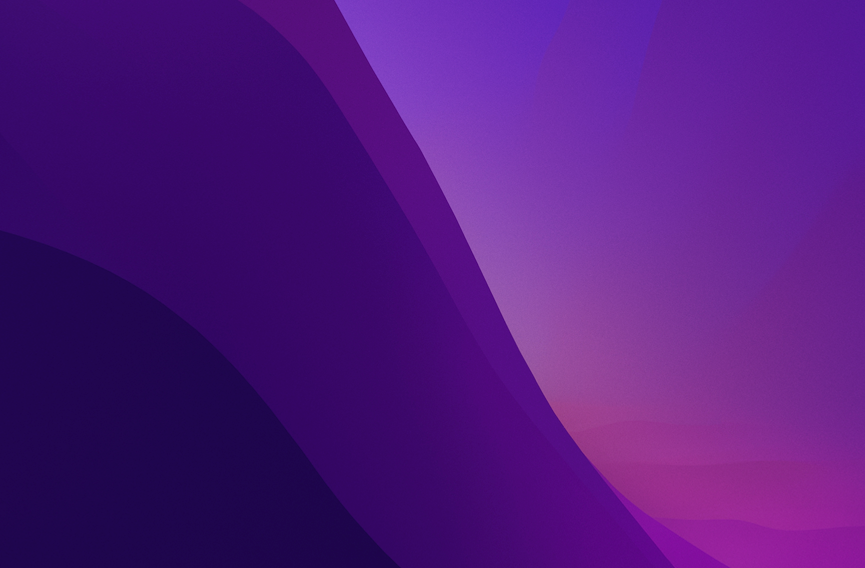
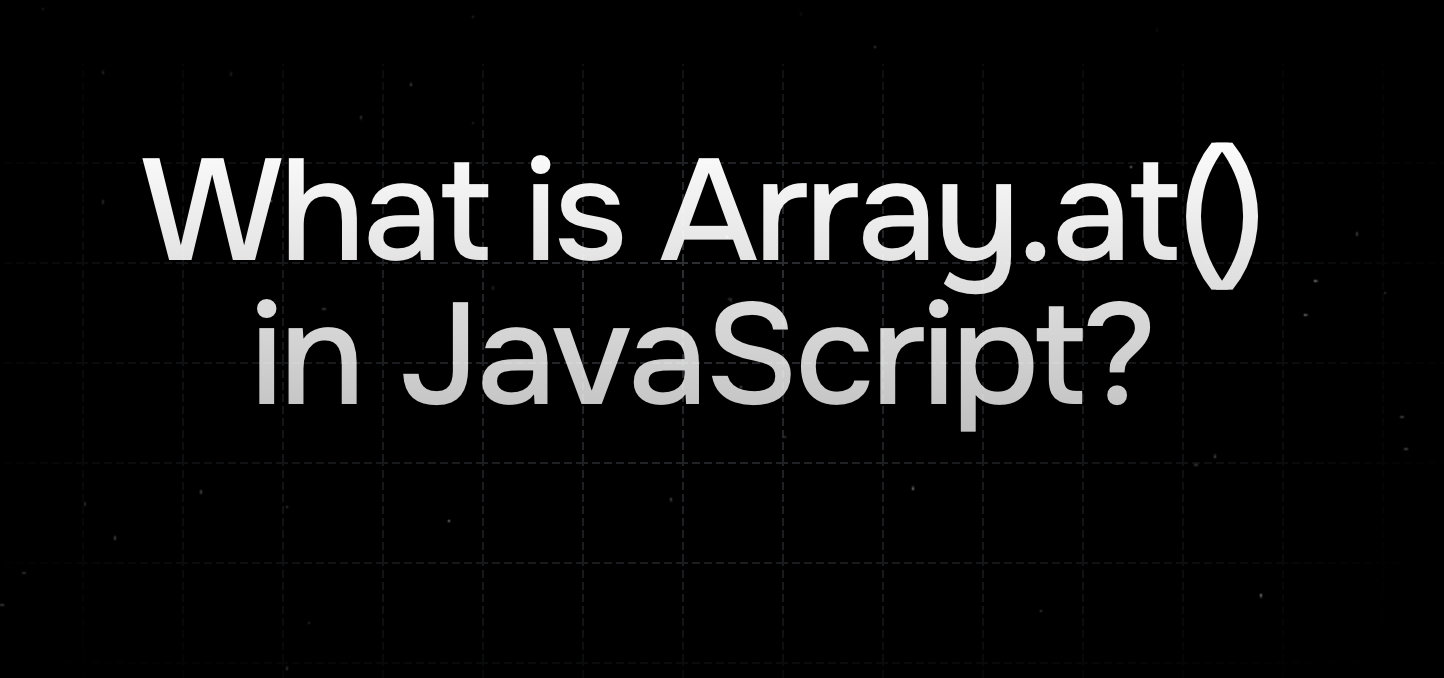
What is array.at() in JavaScript?
Discover how array.at() makes working with arrays in JavaScript more intuit...
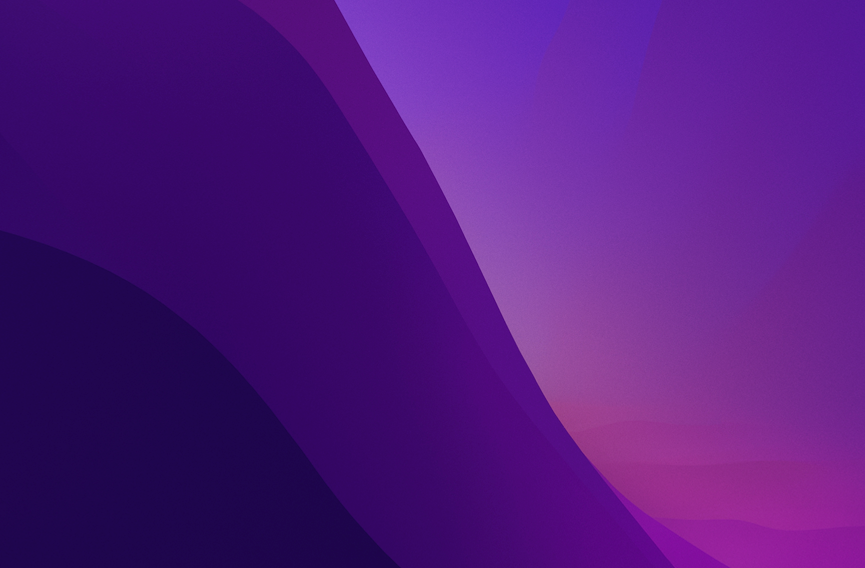
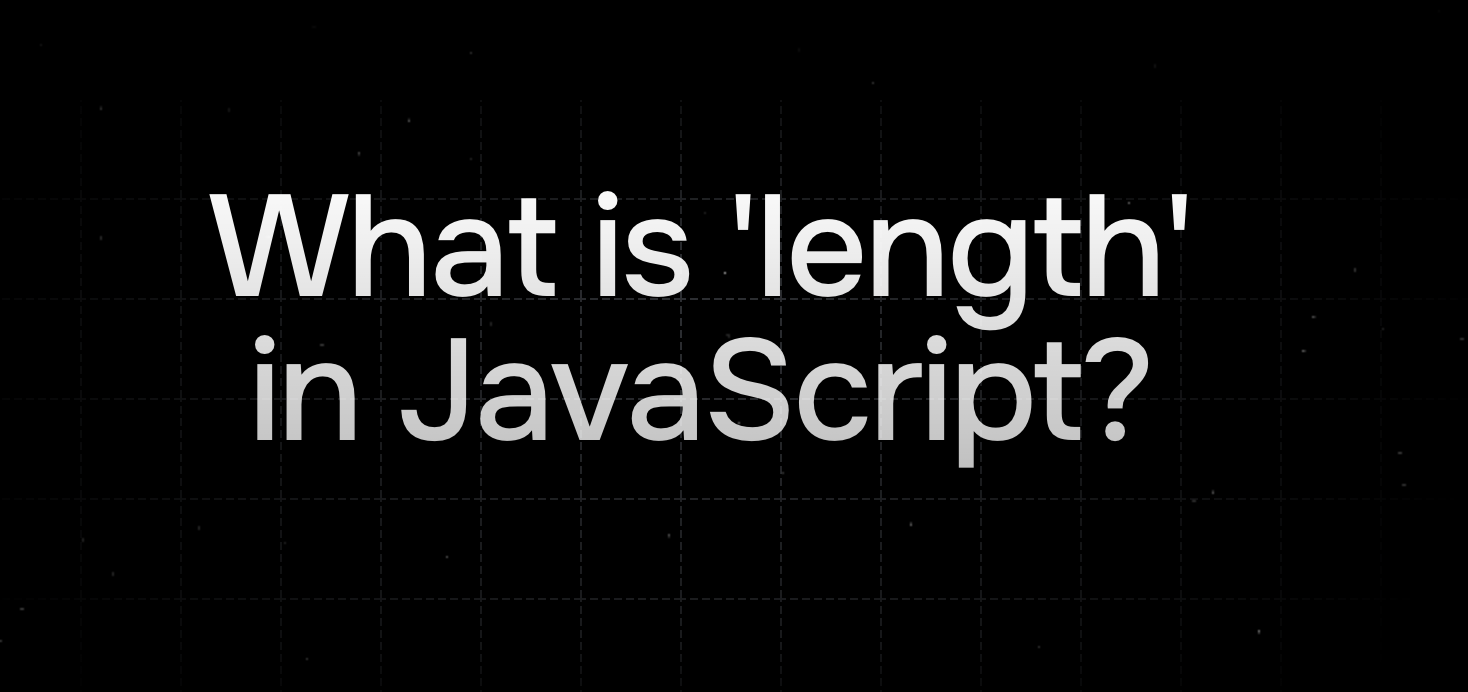
What is length in JavaScript?
Learn how to effectively use JavaScript's length property to work with stri...
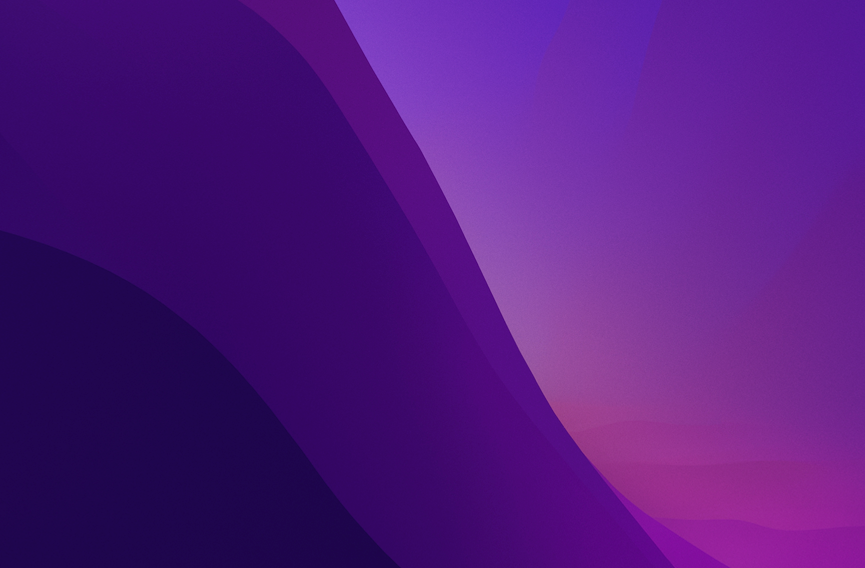