How to use split() in JavaScript
Understand how to use split() in JavaScript to break strings into arrays for easier text manipulation and processing.
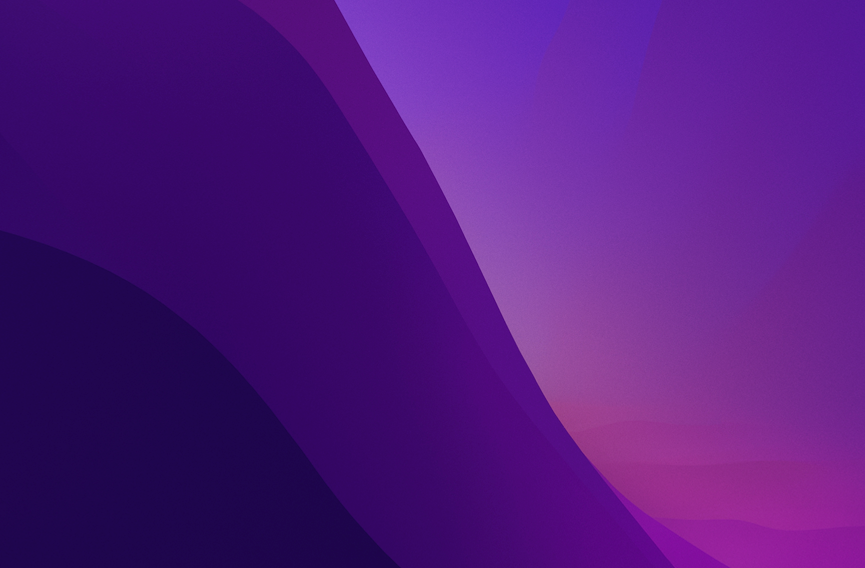
What is split() in JavaScript?
The split() method is a powerful string manipulation tool in JavaScript that transforms strings into arrays based on a specified delimiter. Whether you're parsing CSV data, processing user input, or breaking down complex text structures, split() provides an elegant solution for converting strings into manageable array elements. Let's dive into how this versatile method can simplify your text processing tasks.
1// Basic string splitting2const sentence = "Hello World";3console.log(sentence.split(" ")); // ["Hello", "World"]45// Empty delimiter splits into characters6const word = "JavaScript";7console.log(word.split("")); // ["J", "a", "v", "a", "S", "c", "r", "i", "p", "t"]
The split() method takes a delimiter as its argument and returns an array of substrings. The delimiter can be:
- A simple string
- An empty string (to split into individual characters)
- A regular expression
- Omitted (to return the entire string as a single array element)
💡 Pro tip: Use split() when you need to break down strings into meaningful chunks or convert structured text data into arrays for processing.
1// Different ways to use split()2const csvData = "John,Doe,30,New York";3const dataArray = csvData.split(","); // ["John", "Doe", "30", "New York"]45// Split with limit6const limitedSplit = csvData.split(",", 2); // ["John", "Doe"]78// Split by multiple characters9const text = "apple;banana:orange,grape";10const fruits = text.split(/[;:,]/); // ["apple", "banana", "orange", "grape"]
Let's break down how split() works:
-
Basic splitting:
- Specify a delimiter
- Returns an array of substrings
- Original string remains unchanged
-
Advanced features:
- Optional limit parameter
- Regular expression support
- Handles empty strings
For example:
1// Common split() patterns2const date = "2024-02-06";3const [year, month, day] = date.split("-"); // Destructuring with split45const fullName = "John Smith";6const [firstName, lastName] = fullName.split(" "); // Name parsing78const tags = "javascript,coding,web,development";9const tagArray = tags.split(","); // Creating tag arrays
split() is particularly useful in these scenarios:
- Parsing CSV or similar structured data
- Breaking down strings into arrays for processing
- Extracting specific parts of formatted strings
- Converting strings to arrays for manipulation
Let's explore some real-world examples where split() proves invaluable.
Example 1 - Basic Usage
Let's look at common scenarios where split() helps process text data. This method is especially useful when working with structured text formats or user input.
1// Basic split() usage2const emailAddress = "[email protected]";3const [username, domain] = emailAddress.split("@");45// Processing CSV data6const csvLine = "John,Doe,30,Developer";7const [firstName, lastName, age, occupation] = csvLine.split(",");89// Splitting sentences10const paragraph = "This is a sentence. This is another one.";11const sentences = paragraph.split(". ");
These examples demonstrate how split() can handle various text formats, from simple email addresses to more complex data structures. The method's flexibility allows it to adapt to different delimiter patterns while maintaining clean, readable code.
Example 2 - Using Regular Expressions
Regular expressions with split() provide powerful pattern matching capabilities for more complex string splitting scenarios.
1// Advanced splitting with regex2const text = "apple;banana:orange,grape";3const fruits = text.split(/[;:,]/); // Split on multiple delimiters45// Splitting with whitespace6const sentence = "This has irregular spacing";7const words = sentence.split(/\s+/); // ["This", "has", "irregular", "spacing"]89// Capturing delimiters10const data = "value1::value2--value3";11const values = data.split(/(::|--)/); // Includes delimiters in result
Regular expressions enhance split()'s capabilities by allowing complex pattern matching. This is particularly useful when dealing with inconsistent or complex string formats that require flexible splitting rules.
Example 3 - Common Use Cases
Real-world applications often involve processing structured text data. Here are some practical examples of how split() can handle common scenarios.
1// URL parsing2const url = "https://example.com/path?param=value";3const [protocol, rest] = url.split("://");4const [domain, path] = rest.split("/", 2);56// Name formatting7function formatName(fullName) {8 const [firstName, ...rest] = fullName.split(" ");9 const lastName = rest.join(" ");10 return { firstName, lastName };11}1213// Query string parsing14function parseQueryString(queryString) {15 return queryString16 .split("&")17 .map(pair => pair.split("="))18 .reduce((params, [key, value]) => ({19 ...params,20 [key]: value21 }), {});22}
These examples show how split() can be combined with other array methods to create powerful text processing utilities. The URL parsing example demonstrates how to break down complex strings into meaningful parts, while the query string parser shows how to convert structured text into useful data objects.
Example 4 - Error Handling
Robust error handling is essential when working with split(). Here's how to handle common edge cases and potential issues.
1// Safe string splitting2function safeSplit(str, delimiter) {3 if (typeof str !== 'string') {4 return [];5 }6 return str.split(delimiter);7}89// Handling empty strings10const emptyString = "";11console.log(emptyString.split(",")); // [""]1213// Dealing with missing delimiters14const text = "no delimiter here";15console.log(text.split("|")); // ["no delimiter here"]
Error handling ensures your code remains robust when dealing with unexpected input. The safeSplit function demonstrates how to protect against non-string inputs, while the other examples show split()'s behavior with edge cases.
Example 5 - Performance Considerations
Understanding performance implications helps make informed decisions about using split(). Here's a look at performance considerations and alternatives.
1// Performance comparison2const longString = "a,b,c,d,e,f,g".repeat(1000);34console.time('split');5const arr1 = longString.split(",");6console.timeEnd('split');78// Alternative for character counting9console.time('manual');10const charCount = {};11for (let char of longString) {12 charCount[char] = (charCount[char] || 0) + 1;13}14console.timeEnd('manual');
While split() is generally efficient, consider alternatives for very large strings or when only partial processing is needed. For simple character counting or pattern matching, other string methods might be more appropriate.
Practice Questions
Test your understanding of split() with these practice questions.
JavaScript Word Counter Not Handling Multiple Spaces and Punctuation Correctly
Additional Resources
- MDN Web Docs - String.prototype.split()
- JavaScript.info - String methods
- Regular Expressions in JavaScript
Common Interview Questions
- What's the difference between split() and split('')?
- How does split() handle regular expressions?
- What happens when split() is used with an empty string?
Remember: split() is an essential tool for string manipulation in JavaScript, especially when working with structured text data!
Learn to code, faster
Join 650+ developers who are accelerating their coding skills with TechBlitz.