What is Git Branch? - A Complete Guide to Git Branching
Learn everything about Git branches - from basic concepts to advanced branching strategies. Master Git branching with practical examples and best practices.
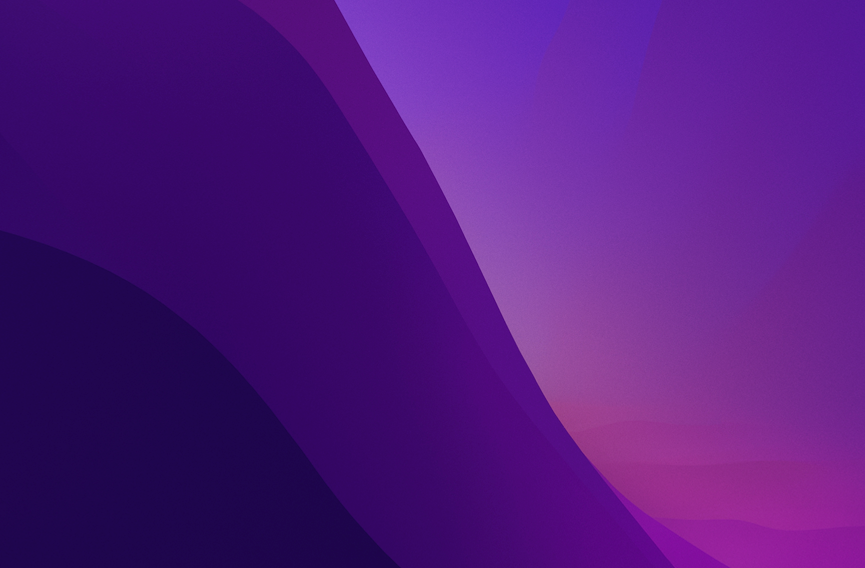
What is a Git Branch?
A Git branch is a lightweight, movable pointer to a commit that allows you to develop features, fix bugs, and experiment with new ideas in isolation from your main codebase. Branches are one of Git's most powerful features, enabling parallel development and efficient collaboration in software projects.
1# View all branches2git branch34# Create a new branch5git branch feature-login67# Switch to the new branch8git checkout feature-login910# Create and switch in one command11git checkout -b feature-login
Think of branches like parallel universes of your code. Each branch maintains its own version of the project, allowing you to:
- Work on new features without affecting the main code
- Experiment with different solutions
- Collaborate with team members without interference
- Maintain multiple versions of your software
💡 Pro tip: Always create a new branch for each feature or bug fix to keep your work organized and isolated.
1# List all branches2git branch34# Create a new branch5git branch <branch-name>67# Switch to a branch8git checkout <branch-name>910# Create and switch (shorthand)11git checkout -b <branch-name>1213# Delete a branch14git branch -d <branch-name>
Common Branching Strategies:
-
Feature Branching
- Create a branch for each new feature
- Merge back to main when complete
- Keeps features isolated during development
-
GitFlow
- Main branch for production code
- Develop branch for ongoing development
- Feature branches for new features
- Release branches for version preparation
- Hotfix branches for urgent fixes
-
Trunk-Based Development
- Short-lived feature branches
- Frequent merges to main
- Emphasis on continuous integration
Creating and Switching Branches
Let's look at a practical example:
1# Start a new feature2git checkout -b feature/user-authentication main34# Make some changes5git add .6git commit -m "Add login form"78# Push the new branch9git push -u origin feature/user-authentication
- The command creates a new branch from 'main'
- Changes are committed to the new branch
- The branch is pushed to the remote repository
💡 Pro tip: Use descriptive branch names that reflect the feature or fix being implemented.
Merging Branches
Here's how to merge your changes back to the main branch:
1# Switch to main branch2git checkout main34# Update main branch5git pull origin main67# Merge your feature branch8git merge feature/user-authentication910# Push the changes11git push origin main
Best practices for merging:
- Always update your main branch first
- Test your changes before merging
- Use meaningful commit messages
- Delete branches after successful merges
💡 Pro tip: Consider using pull requests for better code review and collaboration.
Handling Merge Conflicts
When Git can't automatically merge changes:
1# During a merge conflict2git status # See conflicted files3git diff # View the conflicts45# After resolving conflicts6git add <resolved-files>7git commit -m "Resolve merge conflicts"89# If you need to abort10git merge --abort
Common causes of conflicts:
- Multiple people editing the same file
- Changes to the same lines of code
- Divergent branch histories
Resolution steps:
- Identify conflicted files
- Open and edit the conflicts
- Choose which changes to keep
- Commit the resolution
Branch Management
Best practices for maintaining branches:
1# Clean up old branches2git branch -d feature/completed34# Force delete unmerged branch5git branch -D feature/abandoned67# List merged branches8git branch --merged910# List remote branches11git branch -r
Regular maintenance tasks:
- Delete merged branches
- Review stale branches
- Keep branch names consistent
- Document branch purposes
💡 Pro tip: Regular branch cleanup prevents repository clutter and confusion.
Additional Resources
Common Interview Questions
- What is the difference between git merge and git rebase?
- How do you resolve merge conflicts in Git?
- What is a detached HEAD state?
- How do you recover a deleted branch?
- What is the purpose of the git branch -D command?
Learn to code, faster
Join 650+ developers who are accelerating their coding skills with TechBlitz.
Read related articles
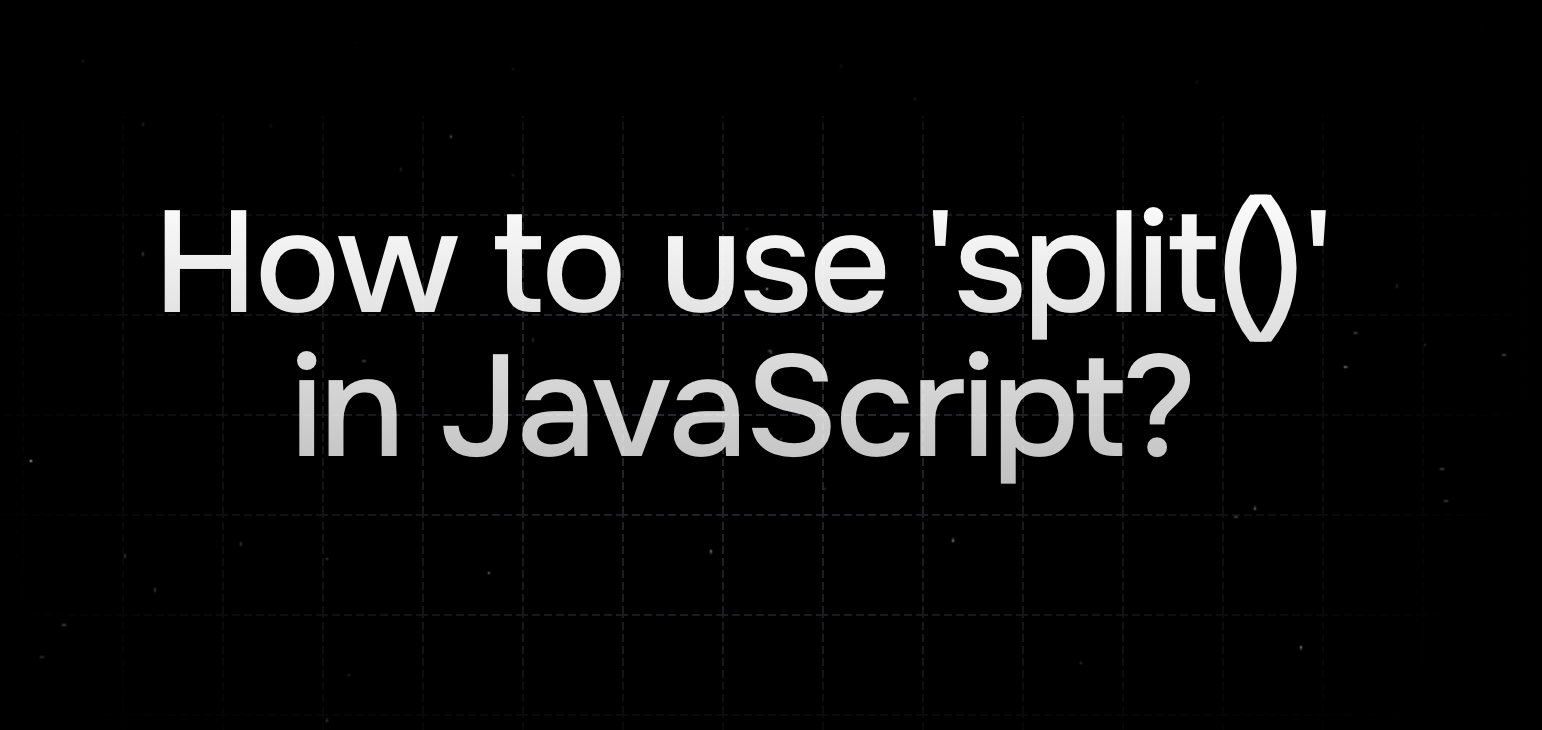
How to use split() in JavaScript
Understand how to use split() in JavaScript to break strings into arrays fo...
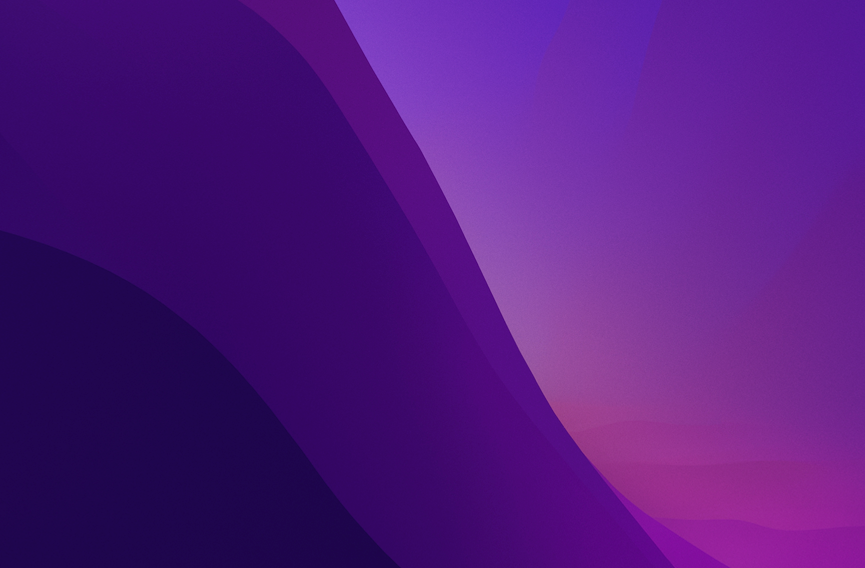
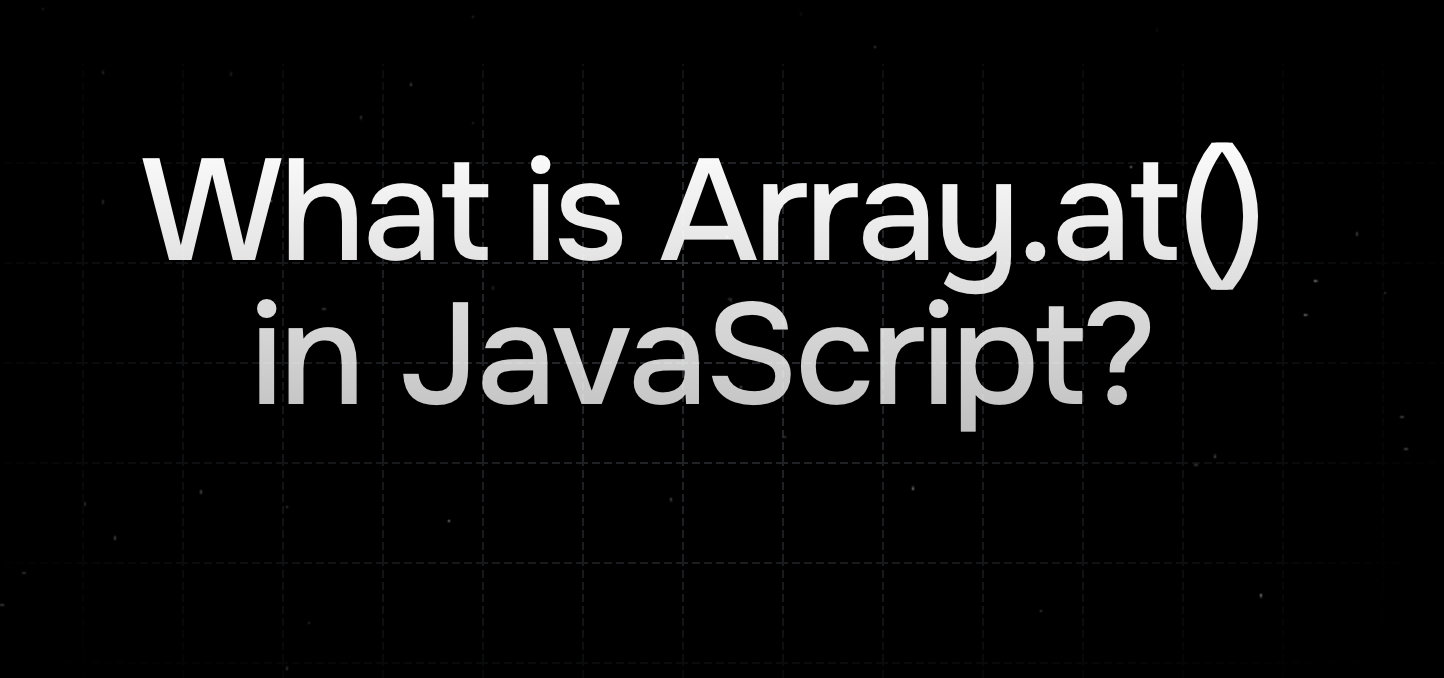
What is array.at() in JavaScript?
Discover how array.at() makes working with arrays in JavaScript more intuit...
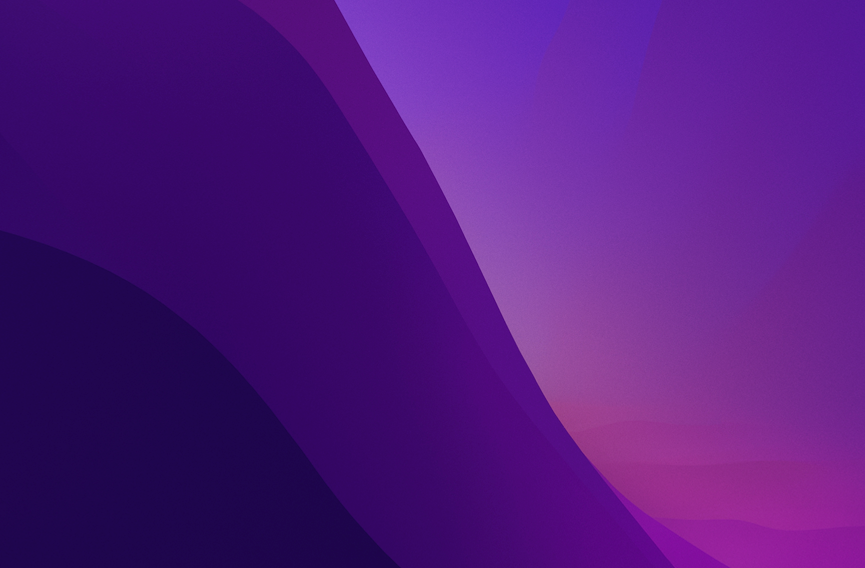
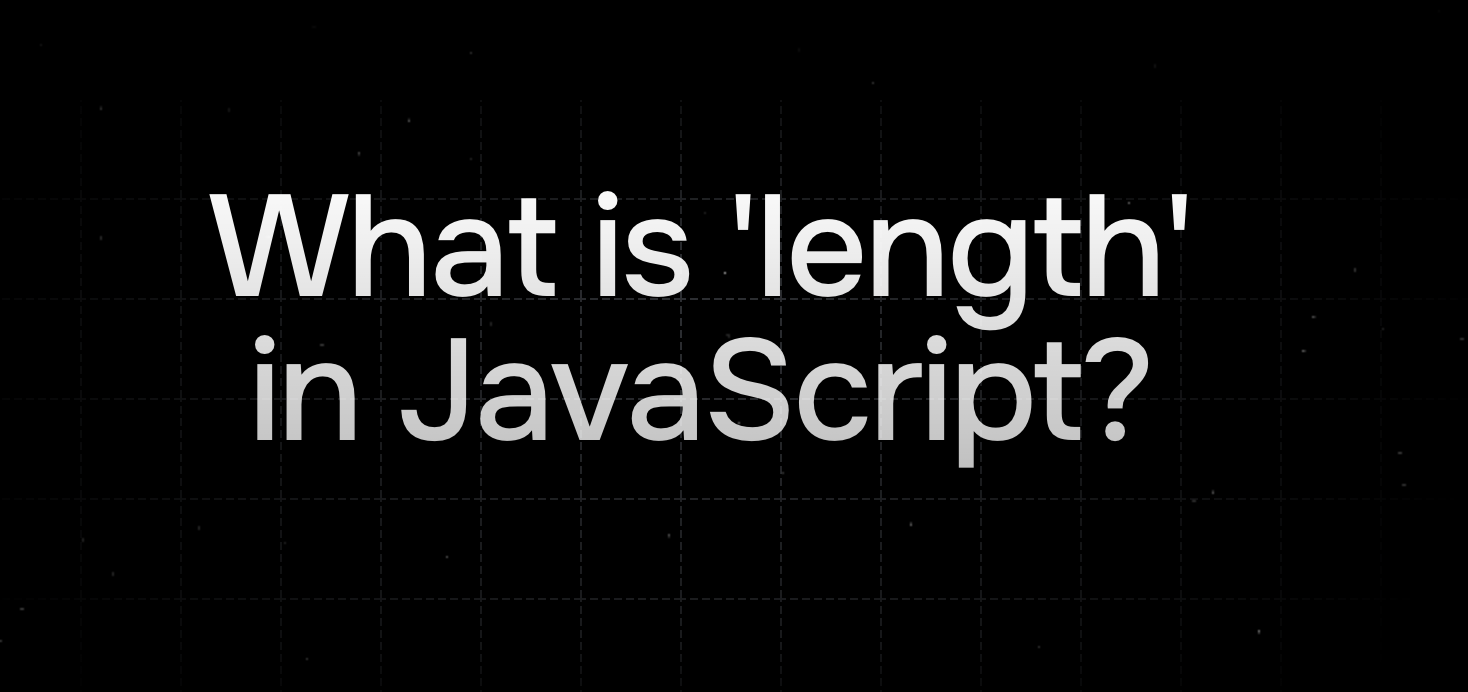
What is length in JavaScript?
Learn how to effectively use JavaScript's length property to work with stri...
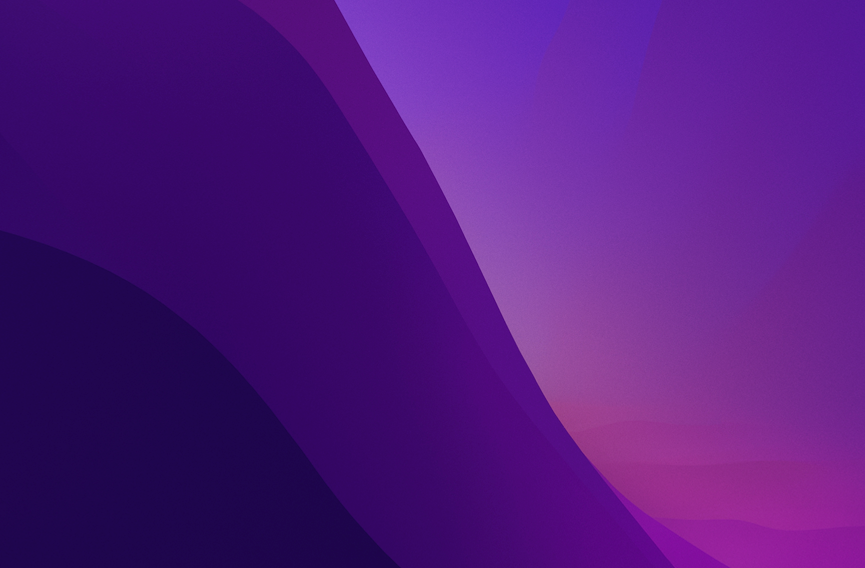