What are Callback Functions in JavaScript - A Complete Guide
Master JavaScript callback functions with clear explanations, practical examples, and real-world use cases. Learn how to write asynchronous code and handle events effectively.
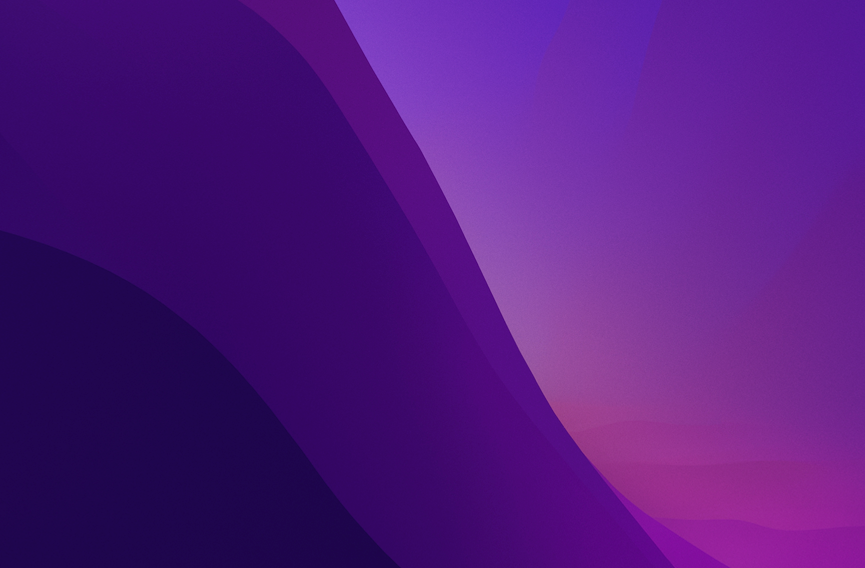
What are Callback Functions?
A callback function is a function that is passed as an argument to another function and is executed after the main function has finished its execution. They are fundamental to JavaScript's asynchronous programming model and are extensively used in event handling, array methods, and working with APIs.
1// Basic callback example2function greet(name, callback) {3console.log('Hello ' + name);4callback();5}67function callbackFunction() {8console.log('Callback function executed!');9}1011greet('John', callbackFunction);12// Output:13// Hello John14// Callback function executed!
In this example, we pass callbackFunction as an argument to the greet function. The callback is executed after the greeting is displayed. This is a simple synchronous callback, but callbacks are most powerful when used with asynchronous operations.
💡 Pro tip: Callbacks are the foundation of asynchronous programming in JavaScript, making it possible to handle time-consuming operations without blocking the main thread.
1// Function that takes a callback2function doSomething(callback) {3// Main function logic4callback();5}67// Anonymous callback function8doSomething(function() {9console.log('Callback executed');10});1112// Arrow function as callback13doSomething(() => {14console.log('Callback executed');15});
Key points about callbacks:
- They can be named functions or anonymous functions
- They can take parameters
- They can be arrow functions
- They're commonly used in asynchronous operations
- They can be nested (though this might lead to callback hell)
Let's explore some real-world examples where callbacks are commonly used.
Example 1 - Event Handling in the Browser
1// Event listener with callback function2const button = document.getElementById('button');34button.addEventListener('click', function(event) {5// event is the Event object containing details about what happened6console.log('Button clicked!');7console.log('Click coordinates:', event.clientX, event.clientY);8console.log('Target element:', event.target);910// We can prevent the default behavior11event.preventDefault();1213// Or stop event propagation14event.stopPropagation();15});1617// Same example with arrow function syntax18button.addEventListener('click', (event) => {19// Arrow functions provide a more concise syntax20// but 'this' behaves differently inside them21console.log('Button clicked!');22console.log('Event type:', event.type);23});
Event handling is one of the most common and important use cases for callbacks in web development. Let's break down how it works:
-
The
addEventListener
method takes two main arguments:- First argument: The event type to listen for (e.g., 'click', 'submit', 'keydown')
- Second argument: The callback function to execute when the event occurs
-
The callback receives an Event object (the
event
parameter) that contains:- Properties describing what happened (coordinates, timestamps, etc.)
- Methods to control event behavior (preventDefault, stopPropagation)
- References to the elements involved (target, currentTarget)
-
This pattern enables:
- Responsive user interfaces
- Decoupled event handling logic
- Multiple handlers for the same event
- Dynamic event handling (add/remove listeners)
Example 2 - Array Methods with Callbacks
1const numbers = [1, 2, 3, 4, 5];23// forEach example - executing code for each element4console.log('forEach example:');5numbers.forEach(function(number, index, array) {6// number: current element7// index: current index8// array: the original array9console.log(`Element ${number} at index ${index}`);10});1112// map example - transforming each element13console.log('\nmap example:');14const doubled = numbers.map((number, index) => {15console.log(`Processing ${number} at index ${index}`);16return number * 2;17});18console.log('Doubled array:', doubled); // [2, 4, 6, 8, 10]1920// filter example with complex condition21console.log('\nfilter example:');22const evenNumbersGreaterThanTwo = numbers.filter((number) => {23const isEven = number % 2 === 0;24const isGreaterThanTwo = number > 2;25console.log(`${number} is even: ${isEven}, > 2: ${isGreaterThanTwo}`);26return isEven && isGreaterThanTwo;27});28console.log('Filtered numbers:', evenNumbersGreaterThanTwo); // [4]
Array methods with callbacks are powerful tools for data transformation. Let's examine each method:
-
forEach
:- Executes a callback for each array element
- Cannot be stopped (unlike for loops)
- Returns undefined
- Perfect for side effects like logging or DOM updates
-
map
:- Creates a new array with transformed elements
- Must return a value in the callback
- Maintains the same array length
- Great for data transformation
- Read more about map here
-
filter
:- Creates a new array with elements that pass the test
- Callback must return true/false
- Resulting array may be shorter
- Excellent for data filtering and validation
- Read more about filter here
Example 3 - Asynchronous Operations with Callbacks
1// Fetching data with error handling and loading states2function fetchData(url, successCallback, errorCallback, loadingCallback) {3// Signal that loading has started4loadingCallback(true);56fetch(url)7 .then(response => {8 if (!response.ok) {9 throw new Error(`HTTP error! status: ${response.status}`);10 }11 return response.json();12 })13 .then(data => {14 // Success case15 loadingCallback(false);16 successCallback(data);17 })18 .catch(error => {19 // Error case20 loadingCallback(false);21 errorCallback(error);22 });23}2425// Using the fetchData function26fetchData(27'https://api.example.com/data',28// Success callback29(data) => {30 console.log('Data received:', data);31 updateUI(data);32},33// Error callback34(error) => {35 console.error('Error occurred:', error);36 showErrorMessage(error);37},38// Loading callback39(isLoading) => {40 const loadingSpinner = document.getElementById('loading');41 loadingSpinner.style.display = isLoading ? 'block' : 'none';42}43);4445// Traditional Node.js style callback pattern46function readFileWithCallback(filename, callback) {47fs.readFile(filename, 'utf8', (error, data) => {48 if (error) {49 callback(error, null);50 return;51 }52 callback(null, data);53});54}
Asynchronous operations are where callbacks truly shine. Let's analyze the patterns:
-
Multiple Callback Parameters:
successCallback
: Handles successful operationserrorCallback
: Handles errorsloadingCallback
: Manages loading states
-
Error-First Pattern (Node.js style):
- First parameter is always the error object
- Second parameter is the success data
- Widely used in Node.js ecosystem
-
Benefits:
- Non-blocking operations
- Proper error handling
- Loading state management
- Flexible response handling
Example 4 - Custom Higher-Order Functions with Callbacks
1// Creating a custom retry mechanism2function retryOperation(operation, maxAttempts, delay, callback) {3let attempts = 0;45function attempt() {6 attempts++;7 console.log(`Attempt ${attempts} of ${maxAttempts}`);89 try {10 const result = operation();11 callback(null, result);12 } catch (error) {13 console.error(`Attempt ${attempts} failed:, error`);1415 if (attempts < maxAttempts) {16 console.log(`Retrying in ${delay}ms...`);17 setTimeout(attempt, delay);18 } else {19 callback(new Error('Max attempts reached'), null);20 }21 }22}2324attempt();25}2627// Using the retry function28retryOperation(29// Operation to retry30() => {31 if (Math.random() < 0.8) throw new Error('Random failure');32 return 'Success!';33},343, // maxAttempts351000, // delay in ms36(error, result) => {37 if (error) {38 console.error('Final error:', error);39 } else {40 console.log('Final result:', result);41 }42}43);
Best Practices:
- Keep callbacks simple and focused
- Use meaningful names for callback parameters
- Handle errors appropriately
- Avoid deeply nested callbacks (callback hell)
- Consider using Promises or async/await for complex async operations
- Document expected callback parameters and behavior
💡 Pro tip: When dealing with multiple asynchronous operations, consider using Promise.all() or async/await instead of nested callbacks to maintain code readability.
Practice Questions
Below are some practice coding challenges to help you master callback functions in JavaScript.
How to Add a Callback Function to Calculate Sum in JavaScript
Additional Resources
Common Interview Questions
- What is a callback function and why would you use one?
- What is callback hell and how can you avoid it?
- How do callbacks relate to asynchronous programming?
- What's the difference between synchronous and asynchronous callbacks?
- How do Promises improve upon traditional callbacks?
Keep practicing with callbacks - they're essential to understanding how JavaScript handles asynchronous operations and event-driven programming!
Learn to code, faster
Join 650+ developers who are accelerating their coding skills with TechBlitz.
Read related articles
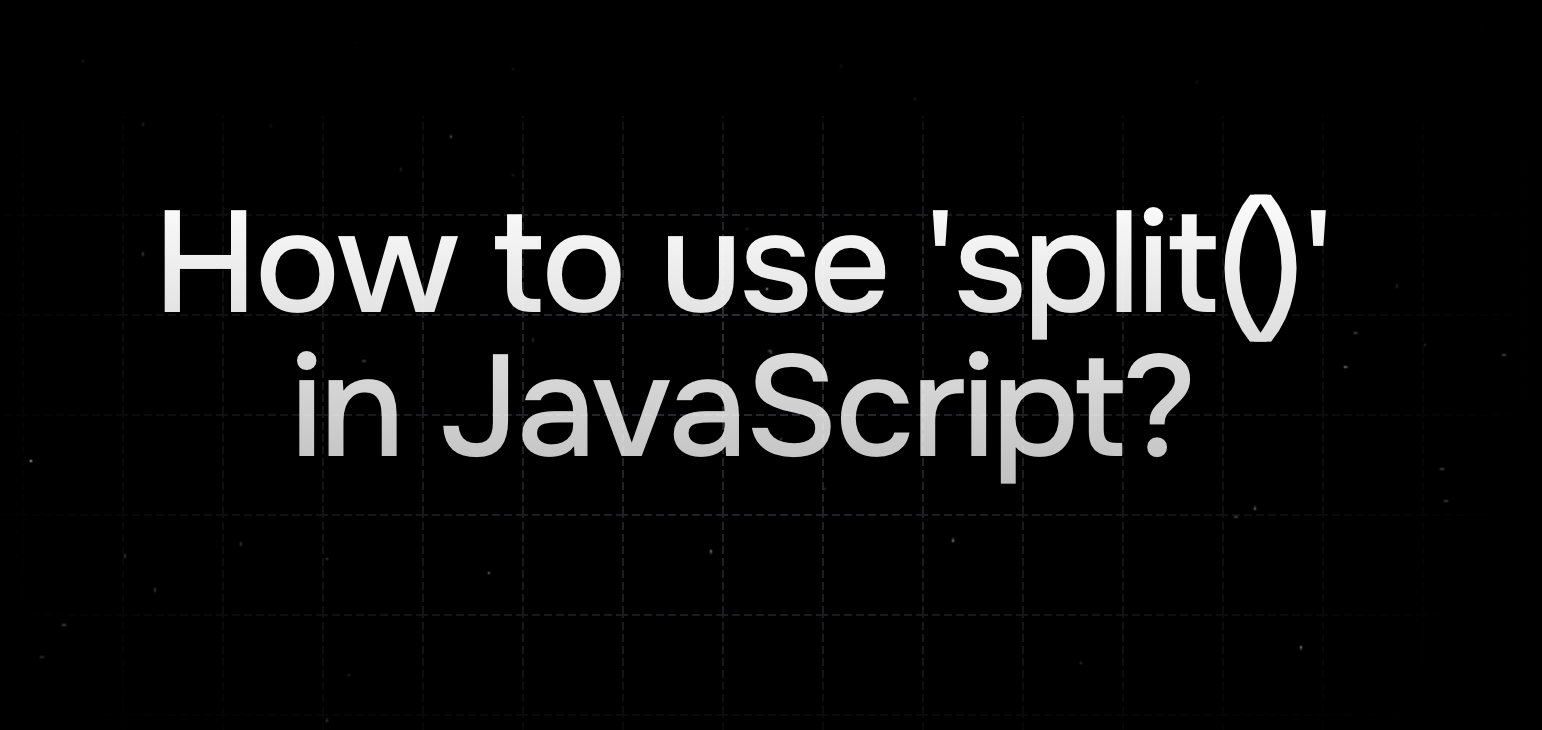
How to use split() in JavaScript
Understand how to use split() in JavaScript to break strings into arrays fo...
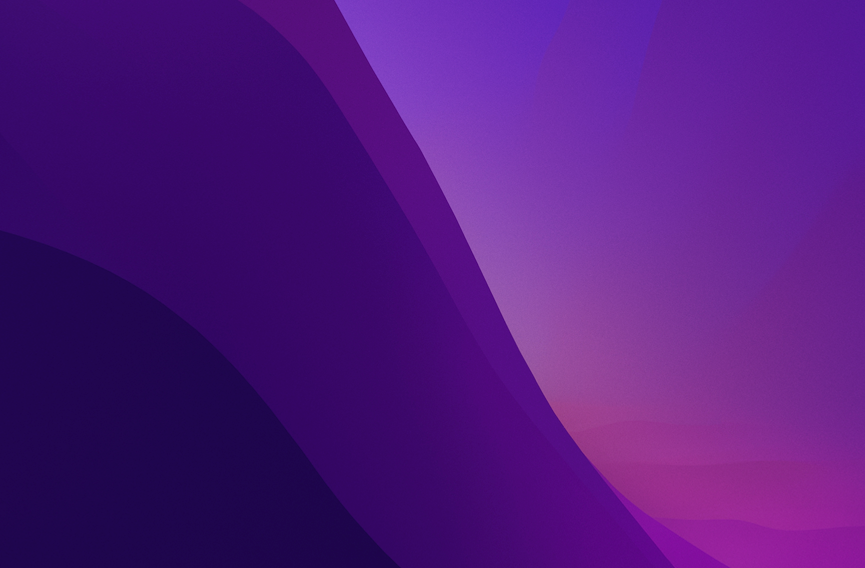
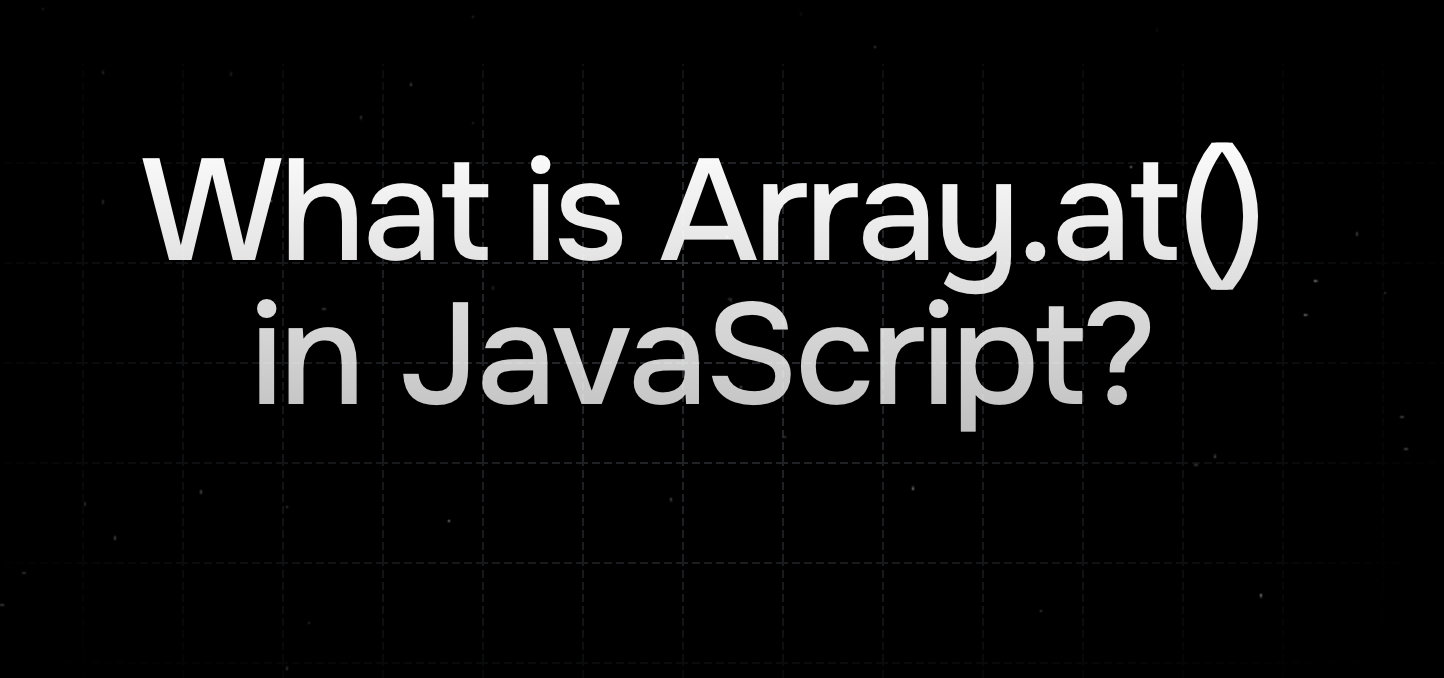
What is array.at() in JavaScript?
Discover how array.at() makes working with arrays in JavaScript more intuit...
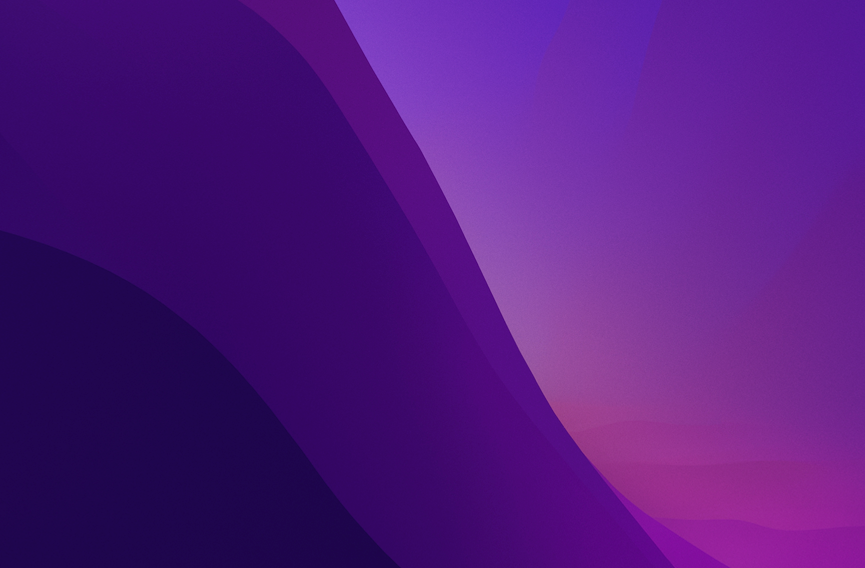
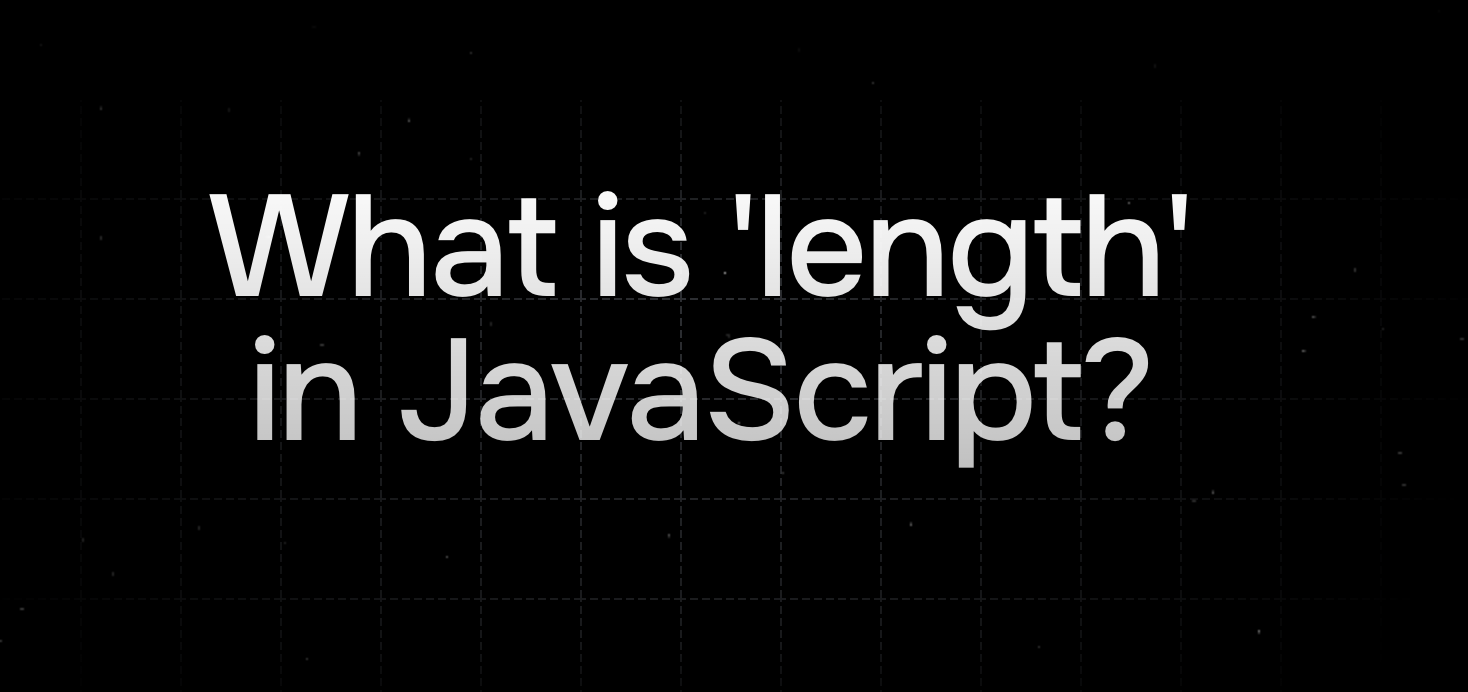
What is length in JavaScript?
Learn how to effectively use JavaScript's length property to work with stri...
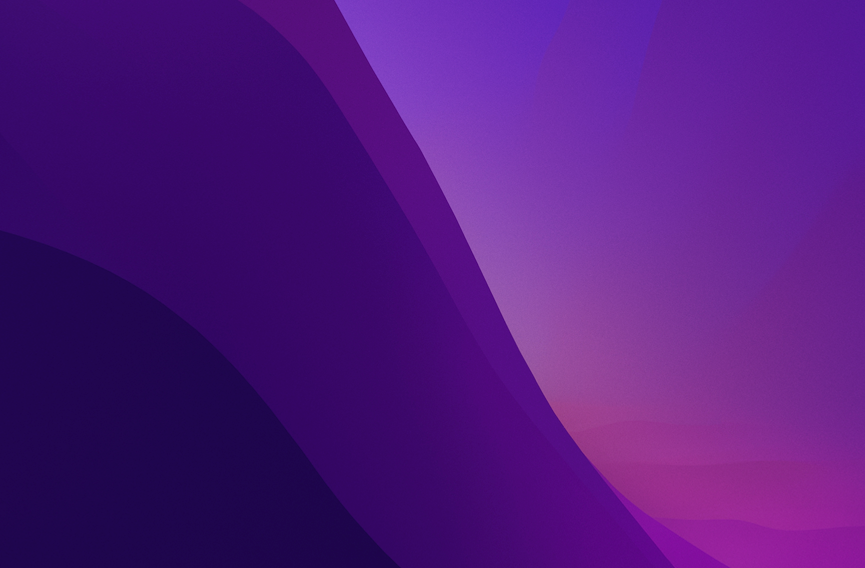