JavaScript Regular Expression Cheat Sheet
Master JavaScript regular expressions with this comprehensive cheat sheet. Learn regular expression methods, syntax, and best practices for working with regular expressions in JavaScript.
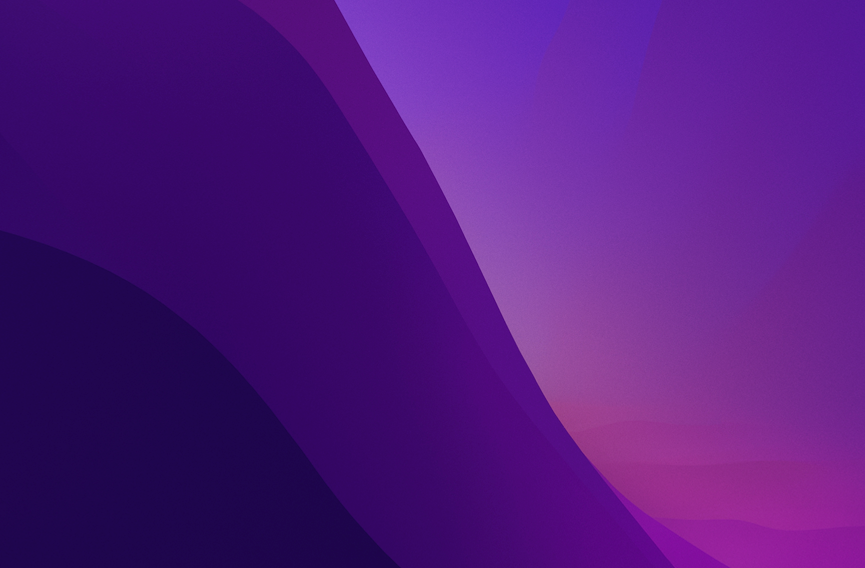
Learn JavaScript: Free JavaScript Course & Regular Expression Cheat Sheet
If you're looking for the best free JavaScript course or a JavaScript online course to improve your skills, you're in the right place. This JavaScript training guide covers essential JavaScript regular expression methods, performance optimization, and common interview questions. Whether you're a beginner looking for a JavaScript beginner course or an advanced developer, this tutorial will help you learn JavaScript online for free!
JavaScript Regular Expressions: The Basics
Regular expressions (regex) are powerful tools for pattern matching and text manipulation in JavaScript. They are widely used for tasks like validation, searching, and replacing text.
Declaring Regular Expressions in JavaScript
You can create regular expressions in two ways:
- Using a regex literal (enclosed in
/
). - Using the
RegExp
constructor.
1// Using regex literal23const regexLiteral = /pattern/;45// Using RegExp constructor67const regexConstructor = new RegExp('pattern');
Common JavaScript Regular Expression Methods
JavaScript provides several methods for working with regular expressions. Mastering these will help you in JavaScript programming classes and real-world projects.
Matching Patterns
-
test()
– Checks if a pattern exists in a string. -
exec()
– Returns the match details ornull
.
1const regex = /hello/;23const str = 'hello world';45console.log(regex.test(str)); // true67console.log(regex.exec(str)); // ['hello', index: 0, input: 'hello world']
Replacing Text
replace()
– Replaces matches with a specified string.
1const str = 'Hello, world!';23const newStr = str.replace(/world/, 'JavaScript');45console.log(newStr); // 'Hello, JavaScript!'
Splitting Strings
split()
– Splits a string into an array based on a regex pattern.
1const str = 'apple,banana,grape';23const fruits = str.split(/,/);45console.log(fruits); // ['apple', 'banana', 'grape']
Regular Expression Flags
Flags modify how a regex pattern is interpreted. Common flags include:
-
g
– Global search (find all matches). -
i
– Case-insensitive search. -
m
– Multiline search.
1const regex = /hello/gi;23const str = 'Hello, hello, HELLO!';45console.log(str.match(regex)); // ['Hello', 'hello', 'HELLO']
Example 1 - Basic Pattern Matching
Regular expressions are commonly used for validating input, such as email addresses or phone numbers.
1const emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;23const email = '[email protected]';4567console.log(emailRegex.test(email)); // true
Example 2 - Using Flags
Flags like g
(global) and i
(case-insensitive) make regex more powerful.
1const regex = /hello/gi;23const str = 'Hello, hello, HELLO!';45console.log(str.match(regex)); // ['Hello', 'hello', 'HELLO']
Example 3 - Advanced Pattern Matching
Regular expressions can handle complex patterns, such as extracting data from strings.
1const dateRegex = /(\d{4})-(\d{2})-(\d{2})/;23const date = '2024-02-06';45const match = dateRegex.exec(date);67console.log(match); // ['2024-02-06', '2024', '02', '06']
Example 4 - Error Handling with Regular Expressions
When working with regex, it's important to handle edge cases and invalid patterns.
1const safeRegex = (pattern, str) => {2try {34 const regex = new RegExp(pattern);56 return regex.test(str);78} catch (error) {910 console.error('Invalid regex pattern:', error);1112 return false;13}14};1516console.log(safeRegex('[a-z', 'test')); // Invalid regex pattern
Example 5 - Performance Considerations
Regular expressions can be computationally expensive. Optimize them for better performance.
1// Avoid nested quantifiers23const slowRegex = /(a+)+/;45const fastRegex = /a+/;67console.time('slowRegex');89slowRegex.test('aaaaaaaaaaaaaaaaaaaa!');1011console.timeEnd('slowRegex'); // Slower1213console.time('fastRegex');1415fastRegex.test('aaaaaaaaaaaaaaaaaaaa!');1617console.timeEnd('fastRegex'); // Faster
Practice Questions
Test your understanding of JavaScript regular expressions with these practice questions.
Count the Vowels
How to Implement a JavaScript Data Validator with Custom Rules and Type Checking
How to Validate Email Addresses Using JavaScript Regex Pattern Matching
Additional JavaScript Resources
Looking for more resources to learn JavaScript regular expressions? Check these out:
What is the best website to learn JavaScript?
If you are looking for a free online platform to learn to code, try our free JavaScript tutorial. This JavaScript online class will help you master JavaScript programming step by step.
TechBlitz is the best site to learn JavaScript. Our free course teaches you HTML, CSS, and JavaScript, ensuring you learn the basics and become a better JavaScript developer in no time!
Learn to code, faster
Join 650+ developers who are accelerating their coding skills with TechBlitz.